Getting Started with Variables – Python Self-Paced Video Training at Lammle.com
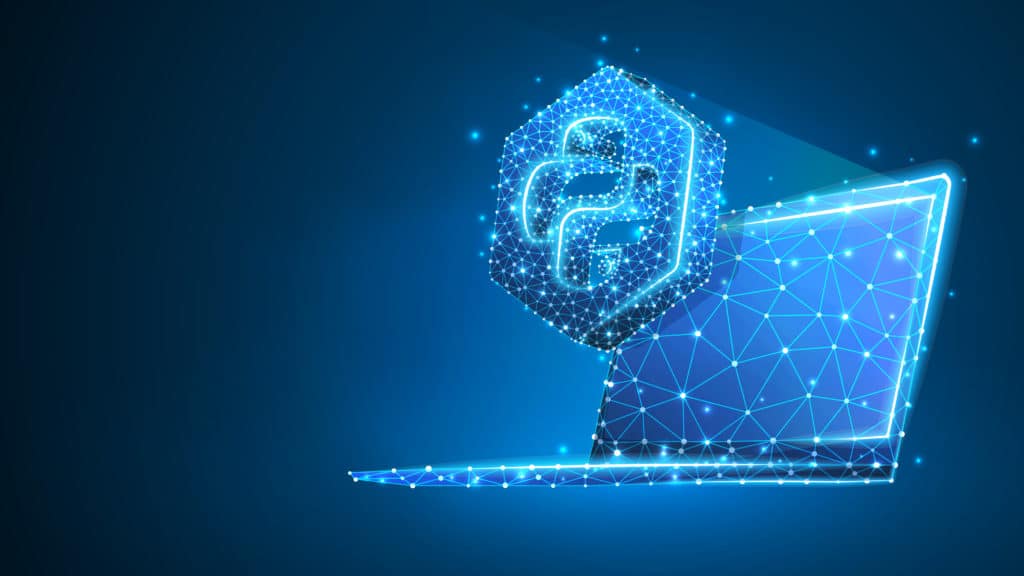
We all know that variables are a key part of programming and scripting languages, and sure enough, they are a big part of Python. Here is how simple they are to implement:
#Today we are going to create and use some variables!
#This is really cool!
#Hey just to remind you, these are comments that are here to make our code “self-documenting”!
my_books = 18
my_years_on_planet = 52
books_per_year_on_planet = my_books / my_years_on_planet
print (“Below is my books per year on the planet!”)
print (books_per_year_on_planet)
Notice here I am using Python 3 so I need parenthesis around the objects and text that I want to print instead of the older quotation marks! This is the most noticeable difference between Python version 2 and version 3. The PRINT function got a nice little update in this regard.
If you are you are using Python 2, you need the “older” syntax to make those print functions work right. Here is that version of our code for you:
#Today we are going to create and use some variables!
#This is really cool!
#Hey just to remind you, these are comments that are here to make our code “self-documenting”!
my_books = 18.0
my_years_on_planet = 52.0
books_per_year_on_planet = my_books / my_years_on_planet
print “Below is my books per year on the planet!”
print books_per_year_on_planet
Notice another change here – I need to indicate my variables are of the Float type in order to display my result in the format I need. So I enter my 14.0 and 47.0 for the variable values. I will discuss this in greater detail in the next post!
One final note, if you wanted to use the print function so that it would work in both version 2 and version 3, you would want to use both the ( and the “ as I did in the first program above. For example, I am using Python 2 now:
print (“Hello World!”)
This returns:
Hello World!
…just as it would in Python 3.
What variable types are supported? THX
Where the Python class information?
Hi Chris, thank you for writing. I was showing a link to a blog post about python variables, but the self paced video FULL course, with hands-on labs directions is at https://www.lammle.com/self-paced-online/
its under Expert Level and can be watched in full with a Platinum license
Thank you!
Todd Lammle